Let's create a game bot using Python
Hello everyone, today we are going to make a bot that will play Piano tiles game.
Piano Tiles is a game where the player’s objective is to tap on the black tiles as they appear from the top of the screen while avoiding the white. When each black tile is tapped, it emits a piano sound.
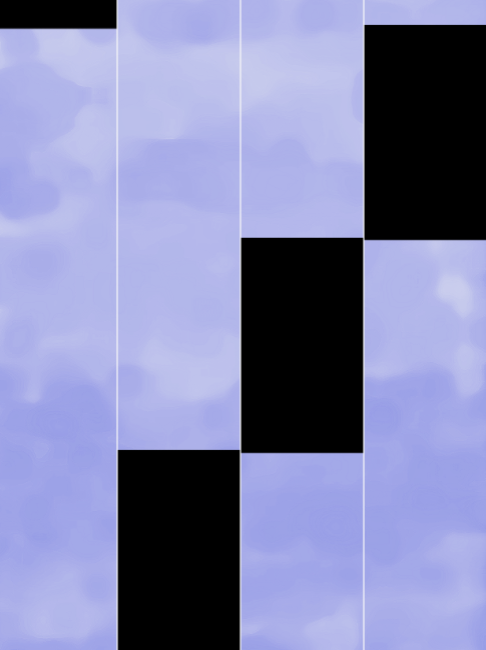
Requirement:
Before we start you need to install these libraries from an administrator terminal (windows):
pip install keyboard
pip install pyautogui
pip install mouse
We also need piano tile game to test our bot. We used this online piano tile game.Â
Bot Logic:
In the Piano tile game, there are usually four columns in which black tiles appear randomly so to make a bot that will play the game, first we need to find the positions of all four columns on the screen. We also want our bot to click in the position when a black tile appears.

we will use two different Python scripts to accomplish the task. The first script will help us in finding the positions of all four columns and the RGB values of the black tile that appears on those columns. I kept the Y position of all columns same(centre of the game screen) as taking different Y positions for all columns is not required.Â
The second script will click if a black tile appears in any of the column positions.
Script to find x and y positions of four columns
import pyautogui
pyautogui.displayMousePosition()
This two-line script will give you the current position of the mouse cursor and the RGB value of the pixel in that position. Move your mouse cursor to every column and note the X positions of each column. Then move the cursor to the middle of the game screen and note the Y position of the mouse.
Also, move your cursor over a black tile and note down the RGB value somewhere(we will be using that).
For my screen resolution these were the positions:
First column X Position: 755
Second column X Position: 903
Third column X Position: 1024
Fourth column X Position: 1155
Middle Screen Y position: 559
RGB value of Black Tile: (0,0,0)
Values will be different for you as it depends on screen resolution.
Script for bot
import time
import keyboard
import pyautogui
import mouse
def click(xPosition,yPosition):
#mouse.move(x,y) moves mouse cursor in given x and y position of your screen
mouse.move(x=xPosition, y=yPosition)
#mouse.press() will press the left mouse button but it will not release it
mouse.press()
# we wait for 0.03 seconds using time.sleep(0.03)
time.sleep(0.03)
#after waiting for 0.03 seconds,
#we will release the mouse click using mouse.release()
mouse.release()
while(keyboard.is_pressed('q')==False):
#755 and 559 is the first column position
if(pyautogui.pixel(755,559)[0]==0):
click(755,559)
#903 and 559 is the second column position
if (pyautogui.pixel(903, 559)[0] == 0):
click(903, 559)
#1024 and 559 is the third column position
if (pyautogui.pixel(1024, 559)[0] == 0):
click(1024, 559)
#1155 and 559 is the fourth column position
if (pyautogui.pixel(1155, 559)[0] == 0):
click(1155, 559)
Let's understand the script:
import time
import keyboard
import pyautogui
import mouse
We are importing the necessary libraries using the above lines of code.
def click(xPosition,yPosition):
#mouse.move(x,y) moves mouse cursor in given x and y position of your screen
mouse.move(x=xPosition, y=yPosition)
#mouse.press() will press the left mouse button but it will not release it
mouse.press()
# we wait for 0.03 seconds using time.sleep(0.03)
time.sleep(0.03)
#after waiting for 0.03 seconds,
#we will release the mouse click using mouse.release()
mouse.release()
We defined a function click(xPosition,yPosition) that takes two values that is x and y position.
We then move the cursor to the given x and y positions using mouse.move(x=xPosition, y=yPosition).
After that we tell the mouse to click on that position using mouse.press()Â but the button will not be released.
We wait for 0.03 secs so that the computer can register the click using time.sleep(0.03). You can make the waiting time smaller if you wish so.
Last but not least, we release the mouse button click using mouse.release().
while(keyboard.is_pressed('q')==False):
if(pyautogui.pixel(755,559)[0]==0):
click(755,559)
if (pyautogui.pixel(903, 559)[0] == 0):
click(903, 559)
if (pyautogui.pixel(1024, 559)[0] == 0):
click(1024, 559)
if (pyautogui.pixel(1155, 559)[0] == 0):
click(1155, 559)
The code will run till ‘q’ is not pressed by the user. This is a failsafe method so that you can stop the bot whenever you want just by pressing the ‘q’ key on your keyboard.
if(pyautogui.pixel(755,559)[0]==0):
click(755,559)
pyautogui.pixel(755,599) returns the RGB value of pixel which has X position as 755 and Y position as 599.If you write this pyautogui.pixel(755,599)[0] then R value is returned and if you typed pyautogui.pixel(755,599)[1] then G value is returned and pyautogui.pixel(755,599)[2] will return B value.
I am only checking if the R-value is 0 just to save some processing time(You can check for G and B values also). If it is true then I am calling the function click(755,599)
while(keyboard.is_pressed('q')==False):
if(pyautogui.pixel(755,559)[0]==0):
click(755,559)
if (pyautogui.pixel(903, 559)[0] == 0):
click(903, 559)
if (pyautogui.pixel(1024, 559)[0] == 0):
click(1024, 559)
if (pyautogui.pixel(1155, 559)[0] == 0):
click(1155, 559)
We check whether R-value is 0 for all column positions and if it is true for any column then we call the function click for that column.
Our bot script is ready. Let’s see how it performs.